15. JavaScript Program to Check given Input is Vowel or Not using Switch Case.
How does this program work?
- In this program you are going to learn about how to Identify the given input is Vowel or Consonant using Switch Case in JavaScript.
Here is the code
<html>
<head>
<title>JavaScript program to Identify the given input is Vowel or Consonant using Switch Case</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter character"/> </td>
</tr>
<tr>
<td> <button onclick = "vowel()">Submit</button> </td>
</tr>
</table>
<div id="num"></div>
</body>
<script type="text/javascript">
function vowel()
{
var ch;
ch = document.getElementById("first").value;
switch(ch)
{
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
case 'A':
case 'E':
case 'I':
case 'O':
case 'U':
document.getElementById("num"). innerHTML= "vowel character";
break;
default: document.getElementById("num"). innerHTML= "Not an vowel";
break;
}
}
</script>
</html>
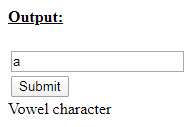
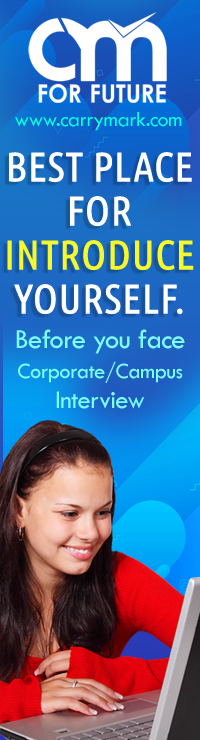