JAVA Theory
Introduction
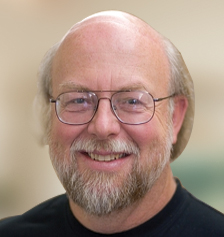
History of JAVA language
Java was started as a project called "Oak" by James Gosling in June 1991. Gosling's goals were to implement a virtual machine and a language that had a familiar C-like notation but with greater uniformity and simplicity than C/C++. The first public implementation was Java 1.0 in 1995. Java is a first programming language which provide the concept of writing programs that can be executed using the web Originally designed for small embedded systems in electronic appliances like set-top boxes Firstly, it was called “Greentalk” by James Gosling and file extension Was gt. After that, it was called Oak and was developed as a part of the Green project.
Structure of JAVA Program
Header | import java.util.Scanner |
---|---|
public class | AddSub{ |
public static | void main |
//main method declaration | declaration |
return | return 0; |
Example program :
Applications
- It is used to develop Web application.
- It is used to Support the develop all mobile operating system.
- Java has strong memory management features, it automatically deletes the unused memory.
Keywords
There are only 50 keywords available in 'JAVA'
Abstract | Assert | Boolean | Break | Byte | Case |
Catch | Char | Class | Const | Continue | Default |
Do | Double | Else | Enum | Extends | Final |
Finally | Float | For | Goto | If | Implements |
Import | Instanceof | Long | Native | New | Null |
Package | Private | Protected | Public | Return | Short |
Static | Strictfp | Super | Switch | Synchronized | This |
Throw | Throws | Transient | Try | Void | Volatile |
While | Return |
Arithmetic Operators
The following table shows all the arithmetic operators supported by the JAVA. Assume variable A holds 5 and variable B holds 10 then
Operator | Description | Example |
---|---|---|
+ | Adds two operators | A + B = 15 |
- | Subtracts second operand from the first | A - B = 5 |
* | Multiplies both operators | A * B = 0 |
/ | Divides numerator by de-nominator | B/A = 0.5 |
% | Modulus operators and remainder of after an integer division | B % A = 0 |
++ | Increment operator increases the integer value by one. | A++ = 11 |
-- | Decrement operator decreases the integer value by one. | A-- = 9 |
Logical Operators
Following table shows all the Logical operators supported by the JAVA. Assume variable A holds 1 and variable B holds 0 then
Operator | Description | Example |
---|---|---|
&& | Called Logical AND operator. If the both operands are non-zero. Then the condition becomes true. Subtracts second operand from the first. Multiplies both operands. Divides numerator by denominator. | (A && B) is false. |
|| | Called Logical OR operator. If any of two operands is non-zero. Then the condition becomes true. | (A||B) is true. |
! | Called Logical NOT operator. It is used to reverse the logical state of its operand. If a condition is true. Then logical not operator Will make it false | !(A && B) is true. |
Relational Operators
The following table shows all the Relational operators supported by the JAVA. Assume variable A holds 10 and variable B holds 20 then
Operator | Description | Example |
---|---|---|
&& | Called Logical AND operator. If the both operands are non-zero. Then the condition becomes true. Subtracts second operand from the first. Multiplies both operands. Divides numerator by denominator. | (A && B) is false. |
|| | Called Logical OR operator. If any of two operands is non-zero. Then the condition becomes true. | (A || B) is true. |
! | Called Logical NOT operator. It is used to reverse the logical state of its operand. If a condition is true. Then logical NOT operator Will make it false | !(A && B) is true |