Java Theory
Polymorphism:
-
1. The process of representing one form in multiple forms is known as Polymorphism.
2. Polymorphism means many forms.
Diagram:
real-life-example-of-polymorphism
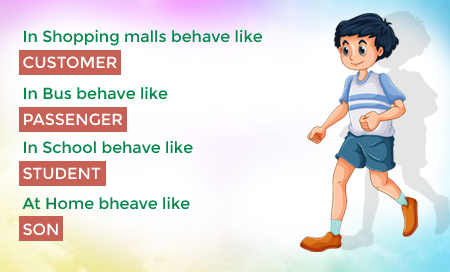
Example of polymorphism:
Polymorphism is the ability to take more than one form. Polymorphism is one of the most important concepts in OOPS ( Object Oriented Programming Concepts). Subclasses of a class can define their own unique behaviors and yet share some of the same functionality of the parent class.
In Java, there are 2 ways by which you can achieve polymorphic behavior:
Overloading
- Same Class, Same Method Name, Different Parameters.Overriding
- Different Class, Same Method Name, Same Parameters.What is Method Overloading in Java?
Method Overloading implies you have more than one method with the same name within the same class but the conditions here is that the parameter which is passed should be different.
Example |
---|
class Overloading { public void disp() { System.out.println("Inside First disp method"); } public void disp(String val) { System.out.println("Inside Second disp method, value is: "+val); } public void disp(String val1,String val2) { System.out.println("Inside Third disp method, values are : "+val1+","+val2); } } public class MethodOverloading_Example { public static void main (String args[]) { Overloading oo = new Overloading(); oo.disp(); //Calls the first disp method oo.disp("Java Interview"); //Calls the second disp method oo.disp("JavaInterview", "Point"); //Calls the third disp method } } |
Example |
---|
The output will be Inside First disp method Inside Second disp method, value is: Java Interview Inside Third disp method, values are : JavaInterview, Point Here the disp() method will be called three times, but the question is how the different disp() are called. The answer is based on the parameter the compiler will choose which methods to be called. |
What is Method Overriding in Java?
Method overriding is almost the same as Method Overloading with a slight change, overriding has the same method name, with the same number of arguments but the methods present in the different classes. Method Overriding is possible only through inheritance.
Example |
---|
class ParentClass { public void disp() { System.out.println("Parent Class is called"); } } class ChildClass extends ParentClass { public void disp() { System.out.println("Child Class is called"); } } public class Overriding_Example { public static void main(String args[]) { ParentClass obj1 = new ParentClass(); //ParentClass reference but ChildClass Object. ParentClass obj2 = new ChildClass(); // Parent Class disp () will be called. obj1.disp(); // Child Class disp () will be called, as it reference to the child class. obj2.disp(); } } |
Example |
---|
Parent Class is called Child Class is called The Child Class disp is called because though the obj2 reference may be a ParentClass reference but the object is a ChildClass object and hence the disp() of the child class is called. This is called as Dynamic Binding or Late Binding or Runtime Polymorphism. |
Use of Super Keyword in Overriding:
If you wish to call the ParentClass method through the Child Class Method then we can use the super keyword to do so. Then the same above example can be re-written as.
Example |
---|
class ParentClass { public void disp() { System.out.println("Parent Class is called"); } } class ChildClass extends ParentClass { public void disp() { super.disp(); System.out.println("Child Class is called"); } } public class Overriding_Example { public static void main(String args[]) { //ParentClass reference but ChildClass Object. ParentClass obj1 = new ChildClass(); // Child Class disp () will be called, as it references the child class. obj1.disp(); } } Here super.disp() will help you call the Parent Class disp(). |
Example |
---|
OutputParent Class is called Child Class is called |