Java Theory
Object:
The Object is the real-time entity having some state and behavior. In Java, Object is an instance of the class having the instance variables like the state of the object and the methods as the behavior of the object. The object of a class can be created by using the new keyword in Java Programming language.
-
1. An object is a real-world entity.
2. An object is a runtime entity.
3. The object is an entity which has state and behavior.
4. The object is an instance of a class.
Example:
1. Dogs have state (name, color, breed, hungry) and behavior (barking, fetching, wagging tail).2. Chair, Bike, Marker, Pen, Table, Car, Book, Apple, Bag etc.
3. It can be physical or logical (tangible and intangible).
Diagram:
Object Example
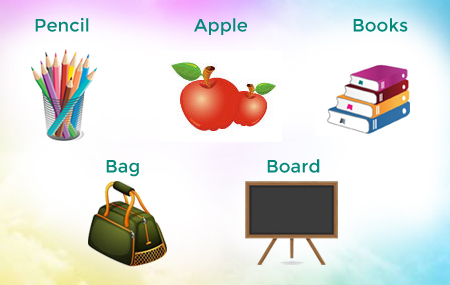
Example |
---|
class Student { int id; //field or data member or instance variable String name; public static void main(String args[]) { Student s1=new Student();//creating an object of Student System.out.println(s1.id);//accessing member through reference variable System.out.println(s1.name); } } |
Creating an Object:
A class provides the blueprints for objects. So basically, an object is created from a class. In Java, the "new" keyword is used to create new objects.
There are three steps when creating an object from a class:
- Declaration − A variable declaration with a variable name with an object type.
- Instantiation − The new keyword is a Java operator that creates the object.
- Initialization − The new operator is followed by a call to a constructor, which initializes the new object.
Example |
---|
public class Puppy { public Puppy(String name) { // This constructor has one parameter, name. System.out.println("Passed Name is :" + name ); } public static void main(String []args) { // Following statement would create an object myPuppy Puppy myPuppy = new Puppy( "tommy" ); } } |