Javascript Theory
String
A string is a combination of characters, which are widely used in JavaScript programming. In javascript strings are treated as objects.
Creating a string:String Declaration
There are two ways to create string.
- String Literal
var str = "Skill Pundit"; - Using New Keyword
var str = new String("SkillPundit");
String length() function
This function is used to find the length of the string.
Example |
---|
var a = "Skillpundit"; var b = a.length(); document.write(b); // Output : 11 |
String compareTo()function
This function is used to compares the given string with current string.
Example |
---|
var a = "Skill"; var b = "Pundit"; var c = "Skill"; document.write(a.compareTo(b)); // Output :greater than zero (because both are not equal) document.write(a.compareTo(c)); // Output : 0(because both are equal) { https://www.tutorialrepublic.com/javascript-reference/javascript-string-object.php } |
String concat() funcion
This function is used to concates of given two strings.
Example |
---|
var a = "Skill"; var b = "Pundit"; var c = a.concat(b); document.write(c); // Output :SkillPundit |
ToLowerCase() function
This function is used to convert the given string characters into lower case characters.
Example |
---|
var a = "SKILLPUNDIT" var b = a.toLowerCase(); document.write(b); // Output : skillpundit |
ToUpperCase() function
This function is used to convert the given string characters into upper case characters.
Example |
---|
var a = "skillpundit" var b = a.toUpperCase(); var c = a.concat(b); document.write(b); // Output : SKILLPUNDIT |
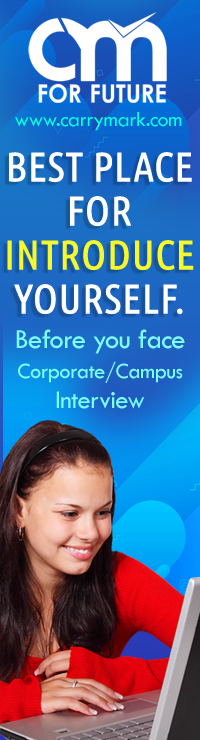