13. JavaScript Program to Determine Whether A Company Insured Driver or Not.
How does this program work?
- In this program you are going to learn about how to Check Status for given problem based on Conditons using JavaScript.
- In this program we can solve the given problem by using nested-if condition.
Here is the code
1. If the driver is married
2. If the driver is unmarried, male and above 30 years of age
3. If the driver is unmarried, female and above 25 years of age
In all other cases, the driver is not insured.
If the marital status, sex, age of the driver are the inputs.
write a program to determine whether the driver is insured or not. (use ‘nested-if’).
<html>
<head>
<title>JavaScript program to Check the Status for the given Problem</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter your marital status (Married/Unmarried)"> </td>
</tr>
<tr>
<td> <input type="text" name="b" id="second" placeholder="Enter your sex (Male/Female)"> </td>
</tr>
<tr>
<td> <input type="text" name="c" id="third" placeholder="Enter your age"> </td>
</tr>
<tr>
<td> <button onclick="conditions ()">Submit</button> </td>
</tr>
</table>
<div id ="num"></div>
</body>
<script type="text/javascript">
function conditions()
{
var a, b, c;
a = document.getElementById("first").value;
b = document.getElementById("second").value;
c = parseInt(document.getElementById("third").value);
if(a == "Married")
{
document.getElementById('num').innerHTML ="Driver is insured";
}
else
if(a == "Unmarried" && b == "Male" && c > 30)
{
document.getElementById ('num').innerHTML = "Driver is insured";
}
else
if(a == "Unmarried" && b == "Female" && c > 25)
{
document.getElementById('num').innerHTML ="Driver is insured";
}
else
{
document.getElementById('num').innerHTML ="Driver is not insured";
}
}
</script>
</html>
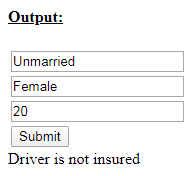
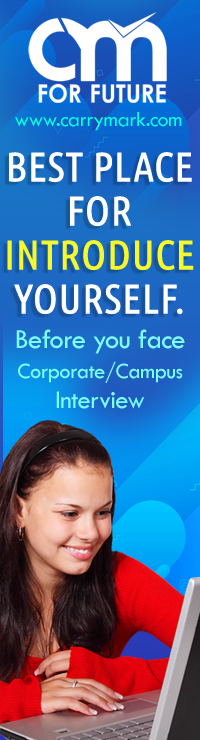