22. JavaScript Program to find Positive numbers, Negative numbers and Zeros of given numbers.
How does this program work?
- In this program we are going to learn about how to find the Positve numbers, Negative numbers and Zeros of given real numbers using JavaScript.
- Declare n variable, array elements stored in this variable.
- Each element is compared with 0, If the element is greater than 0 then the element is postive and the element is less than 0 then the element is negative otherwise the element is zero.
- After doing this for each element of the given array, then we get the postive, negative and zeros numbers of given numbers.
Here is the code
<html>
<head>
<title>JavaScript program to find Positive numbers, Negative numbers and Zeros of given numbers</title>
</head>
<body>
</body>
<script type="text/javascript">
var array = [5, 9, -4, 0, 2, 0, -25, 64, -45, 0 ];
var positive = 0, negative = 0, zero = 0;
for (var i = 0; i < 10; ++i)
{
if (array[i] > 0)
{
positive += 1;
}
else if(array[i] < 0)
{
negative += 1
}
else
{
zero+= 1;
}
}
document.write("Given numbers : "+ array+ "</br>");
document.write("Number of +ve numbers are: "+ positive+"</br>");
document.write("Number of -ve numbers are: "+ negative+"</br>");
document.write("Number of zeros numbers are: "+ zero);
</script>
</html>
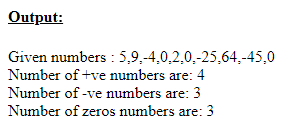
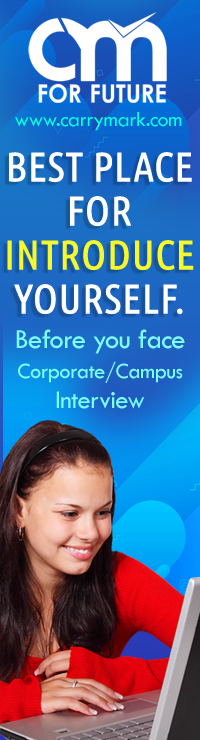