4. JavaScript Program to find Square root of a given number.
How does this program work?
- In this program you will learn about how to do Square root of a given number using JavaScript.
- The following code uses sqrt() mathmetical function.
- And then by using the given logic you will get output. If number is negative, the sqrt function will return a domain error.
Here is the code
<html>
<head>
<title>JavaScript Program to find Square root of a number</title>
</head>
<body>
<table>
<tr>
<td> <input type ="text" name="a" id="first" placeholder="Enter a number"> </td>
</tr>
<tr>
<td> <button onclick = "square()" >Submit</button> </td>
</tr>
</table>
<div id="num"></div>
</body>
<script type="text/javascript">
function square()
{
var a,Square;
a = parseInt(document.getElementById ("first").value);
//To find square root of a number using sqrt() function
Square = Math.sqrt(a);
document.getElementById ("num").innerHTML = "Square Root of "+a+" is : "+Square;
}
</script>
</html>
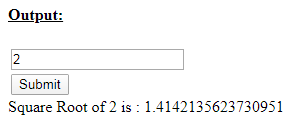
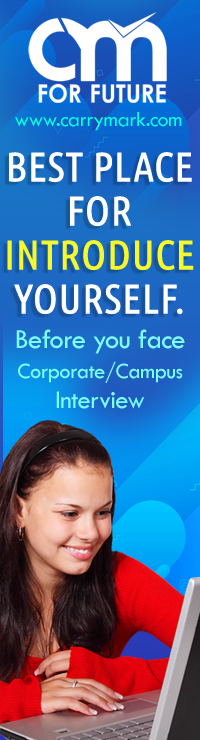