18. JavaScript Program to find the Sum of n distinct numbers.
How does this program work?
- In this program we are going to learn about how to find the Sum of n distinct numbers using JavaScript.
- The integer entered by the user is stored in variable n.
- Declare variable sum to store the sum of numbers and initialize it with 0.
- By using for loop we can add the sum of n distinct numbers.
Here is the code
<html>
<head>
<title>JavaScript program to find the Sum of n distinct numbers</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter distinct number"/> </td>
</tr>
<tr>
<td> <button onclick="sum ()">Submit</button> </td>
</tr>
</table>
<div id="num"></div>
</body>
<script type="text/javascript">
function sum()
{
var n,i, sum = 0;
n = parseInt(document.getElementById ("first").value);
for(i = 1; i <= n; i++)
{
sum = sum+i;
}
document.getElementById ("num").innerHTML="Sum of "+n+ " distinct numbers is :"+sum;
}
</script>
</html>
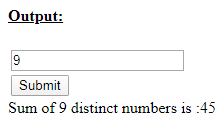
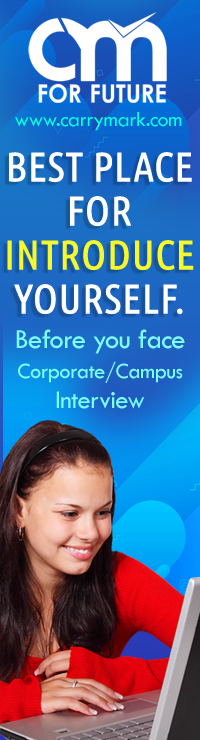