79. JAVA Program to Convert Decimal number into Octal number.
How does this program work?
- This program is used to convert given Decimal number into equivalent of Octal number using Java.
- That means convert number with base value 10 to base value 8.
- The integer entered by user will store in one variable. Divide that number by 8.
- Store the remainder when the number is divided by 8 in an array, Repeat the above step until the number is greater than zero.
Here is the code
// Programme to convert an integer into octal number
import java.util.Scanner;public class Number
{
public static void main(String args[])
{
int dec_num, rem, quot, i=1, j;
int oct_num[] = new int[100];
System.out.print("Enter Decimal Number:\n");
Scanner skill = new Scanner( System.in);
dec_num = skill.nextInt();
quot = dec_num;
while(quot != 0)
{
oct_num[i++] = quot%8;
quot = quot/8;
}
System.out.print("Equivalent Octal number is: ");
for(j=i-1; j>0; j--)
{
System.out.print(oct_num[j] );
}
System.out.print("\n" );
}
}
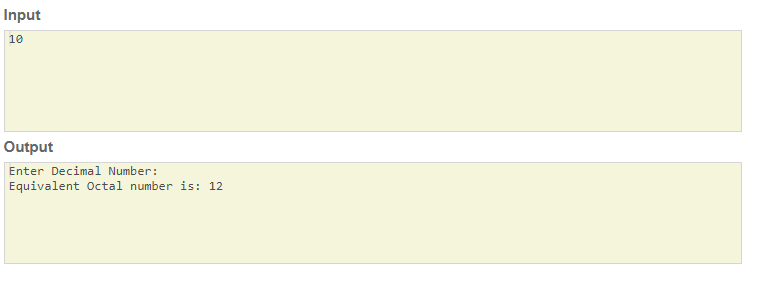