149. JAVA Program to count the number of Vowels, Consonants and Whitespaces of given text.
How does this program work?
- This program is used to count the number of Vowels, Consonants and Whitespaces of a given line of text using java.
- By using if condition we can find the number of vowels, consonants and whitespaces from given text.
Here is the code
//To count the number of Vowels, Consonants and Whitespaces in a given line of text
import java.util.Scanner;public class Count
{
public static void main(String args[] )
{
int i,vowels=0,consonants=0,digits=0,spaces=0,specialCharacters=0;
String s;
System.out.println("Enter sentence" );
Scanner obj = new Scanner(System.in );
s=obj.nextLine();
char str[] = s.toCharArray();
int n= str.length;
for(i=0;i< n;i++)
{
if(str[i]=='a' || str[i]=='e' || str[i]=='i' ||str[i]=='o' || str[i]=='u' || str[i]=='A' || str[i]=='E' || str[i]=='I' || str[i]=='O' || str[i]=='U')
{
vowels++;
}
else
if((str[i]>='a'&& str[i]<='z') || (str[i]>='A'&& str[i]<='Z'))
{
consonants++;
}
else
if(str[i]>='0' && str[i]<='9')
{
digits++;
}
else
if(str[i]==' ')
{
spaces++;
}
else
{
specialCharacters++;
}
}
System.out.println("Vowels = "+vowels);
System.out.println("Consonants ="+consonants);
System.out.println("Digits = "+digits);
System.out.println("White spaces = "+spaces);
System.out.println("Special characters ="+specialCharacters);
}
}
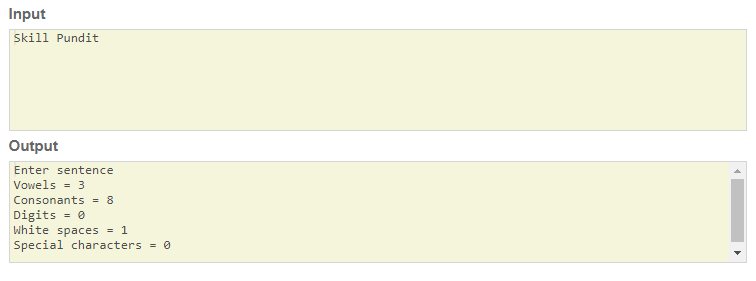