74. JAVA Program to Display frequency of each element from array.
How does this program work?
- This program is used to find frequency of each element in given array using java.
- In this program we need to count the occurrence of each element present in the array.
- Declare two arrays one will be store elements, and another(fr) will be store the frequencies of elements present in an array.
- And variable check will initialize with -1, because it helps us to avoid counting the same element again in the given array.
Here is the code
//To display frequency table of given array elements
public class Frequency{
public static void main(String[] args )
{
//Initialize array
int arr [] = new int [] {3, 2, 5, 3, 2, 4, 2, 5, 3,9,1};int fr[] = new int [arr.length];
int visited = -1;
for(int i = 0; i < arr.length; i++)
{
int count = 1;
for(int j = i+1; j < arr.length; j++)
{
if(arr[i] == arr[j])
{
count++;
fr[j] = visited;
}
}
if(fr[i] != visited)
fr[i] = count;
}
//Displays the frequency of each element present in array
System.out.println(" Element | Frequency");for(int i = 0; i < fr.length; i++)
{
if(fr[i] != visited)
System.out.println(" " + arr[i] + " | " + fr[i]);
}
}
}
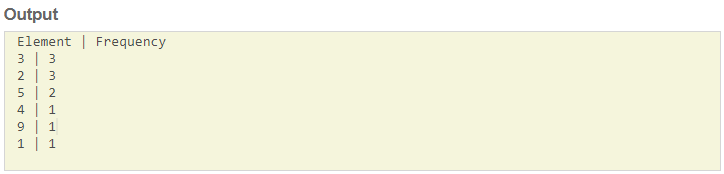