67. JAVA Program to find the Fibonacci series of a given number.
How does this program work?
- This program is used to find the Fibonacci series of a given number using Java.
- Fibonacci series means the previous two elements are added to get the next element starting with 0 and 1.
- First we initializing first and second number as 0 and 1, and print them.
- The third number will be the sum of the first two numbers by using loop.
Here is the code
//To find the Fibonacci series of a given number
import java.util.Scanner;public class Fibonacci
{
public static void main(String[] args)
{
int n, first = 0,next = 1;
System.out.println("Enter number::");
Scanner obj= new Scanner(System.in);
n = obj.nextInt();
System.out.print("The " + n + " Fibonacci numbers are: ");
System.out.print(first + " " + next);
for ( int i = 1; i<=n-2; ++i )
{
int sum = first + next;
first = next;
next = sum;
System.out.print(" " + sum);
}
}
}
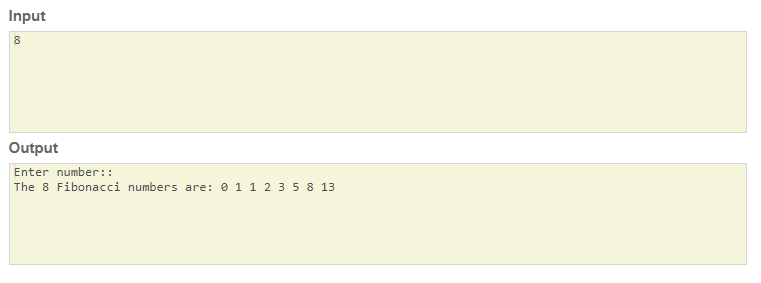