134. JAVA Program to find the Binomial Coefficients using recursion.
How does this program work?
- This program is used to find the Binomial Coefficient using recursion in java.
- Declare variable are to store reverse number and initialize it with 0.
- Multiply the reverse number by 10, add the remainder which comes after dividing the number by 10.
Here is the code
//To find the Binomial Coefficient using recursion
import java.util.Scanner;public class Coefficient
{
public static void main(String args[] )
{
int n,r;
System.out.println("Enter a number N:");
Scanner obj = new Scanner( System.in);
n=obj.nextInt();
System.out.println("Enter a number R:");
r=obj.nextInt();
int ncr=fact(n)/(fact(r)*fact(n-r));
System.out.println("The "+n+"C"+r+" value is: = "+ncr);
}
static int fact(int n)
{
int i,f=1;
for(i=1;i<=n;i++)
{
f=f*i;
}
return f;
}}
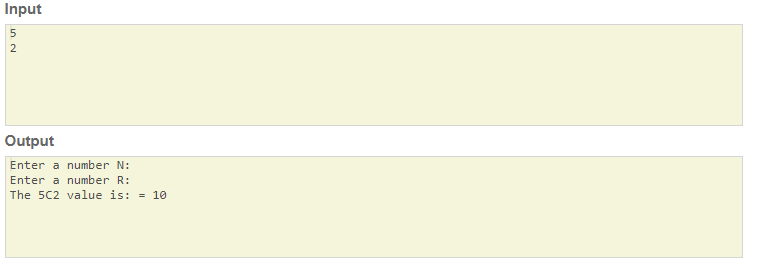