128. JAVA Program to find the Factorial of a given number using recursion.
How does this program work?
- This program is used to find the factorial of a given number using recursion in java.
- Factorial number is defined by the product of all the digits of given number.
- Factorial of 0 is always 1.
- Recursion method is used to find the factorial of a given number.
Here is the code
//To find the Factorial of a given number using recursion
import java.util.Scanner;public class factorial
{
public static void main(String args[] )
{
Scanner obj = new Scanner(System.in );
System.out.println("Enter number:" );
int num = obj.nextInt();
int factorial = fact(num);
System.out.println("Factorial of given number is: "+factorial);
}
static int fact(int n )
{
int output;
if(n==1)
{
return 1;
}//Recursion: Function calling itself!!
output = fact( n-1 ) * n;return output;
}}
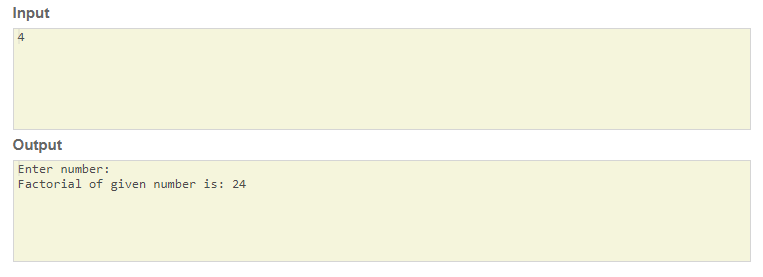