53. JAVA Program to Check if a number is Positive or Negative.
How does this program work?
- In this program you are going to learn about to check if a number is positive or negative using JAVA.
- This problem is solved using if and nested if...else statement.
- A number is positive if it is greater than zero. We check this in the expression of if.
- If it is False, the number will negative.
Here is the code
//Programme to Count positive numbers and negative numbers and zeros in a array.
import java.util.Scanner;public class Number
{
public static void main(String args[])
{
// Intialize and declaring the objects.
int n,positive=0, negative=0, zero=0, i;int arr[] = new int[50];
// Enter number you have to enter.
System.out.print("How many Number you want to Enter:\n ");Scanner skill= new Scanner(System.in );
n = skill.nextInt();
// Enter the numbers.
System.out.println("Enter " +n+ " Numbers : ");// This is to calculate the type of the number.
for(i=0; i< n; i++){
arr[i] = skill.nextInt();
}
for(i=0; i< n; i++)
{
if(arr[i] < 0)
{
negative++;
}
else
if(arr[i] == 0)
{
zero++;
}
else
{
positive++;
}
}
// print all +ve,-ve and zero number.
System.out.println("The count of Positive Numbers are: " + positive );System.out.println("\nThe count of Negative Numbers are: " + negative );
System.out.println("\nThe count of Zeros are: " + zero );
}
}
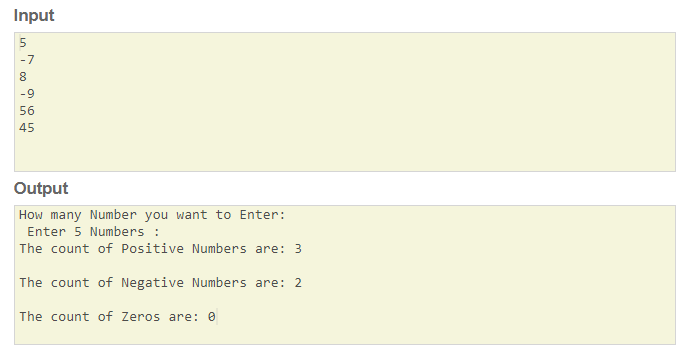