61. JAVA Program to find out the Sum of Even and Product of Odd digits of given 6 digit Number.
How does this program work?
- This program is used to find out the Sum of Even and Product of Odd digits in the given numbers using JAVA.
- In this program odd and even A number is even if it is perfectly divisible by 2.
- When the number is divided by 2, we use the remainder operator % to compute the remainder.
- If the remainder is zero, the number is even then sum will be executes else the number is odd then product will be executed by using given logic.
Here is the code
import java.util.Scanner;
public class sum
{
public static void main(String[] args)
{
int n, even = 0, odd = 0;
System.out.print("Enter the number of elements in array:\n" );
Scanner obj = new Scanner(System.in);
n = obj.nextInt();
int[] a = new int[n];
System.out.println("Enter the elements of the array:\n");
for(int i = 0; i < n; i++)
{
a[i] = obj.nextInt();
}
for(int i = 0; i < n; i++)
{
if(a[i] % 2 == 0)
{
even = even + a[i];
}
else
{
odd = odd + a[i];
}
}
System.out.println("Sum of Even Numbers:"+even);
System.out.println("Sum of Odd Numbers:"+odd);
}
}
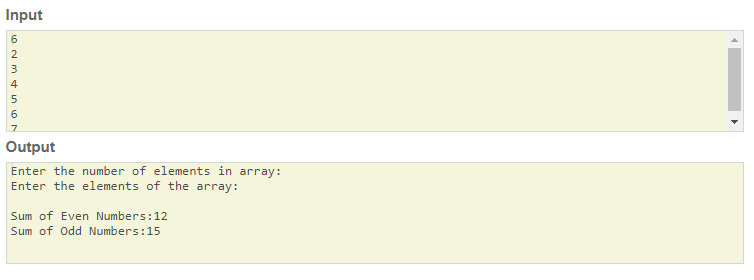