30. JAVA Program To Find The Type of Triangle If 3sides are given.
How does this program work?
- In this program you are going to learn about how to find Type of Triangle using 3sides.
- Entered 3 Sides are Stored 3 Variables a,b,c .
- By using the below if conditions the correct type of Triangle is identified using java.
Here is the code
// Types of Triangles
import java.util.Scanner;public class Triangle
{
public static void main(String args[] )
{
int a,b,c;
System.out.println("Enter the lenghts of 3sides of triangles\n");
Scanner obj=new Scanner(System.in );
a=obj.nextInt();
b=obj.nextInt();
c=obj.nextInt();
if ( (a*a)+(b*b)==(c*c) || (b*b)+(c*c)==(a*a) || (c*c)+(a*a)==(b*b) )
{
System.out.println("Right angle triangle\n");
}
else
if((a==b) && (b==c))
{
System.out.println("Equilateral triangle\n");
}
else
if (( a==b) || (b==c) || (c==a) )
{
System.out.println("Isosceles triangle\n");
}
else
if((a!=b && b!=c && c!=a) )
{
System.out.println("Scalene triangle\n");
}
}
}
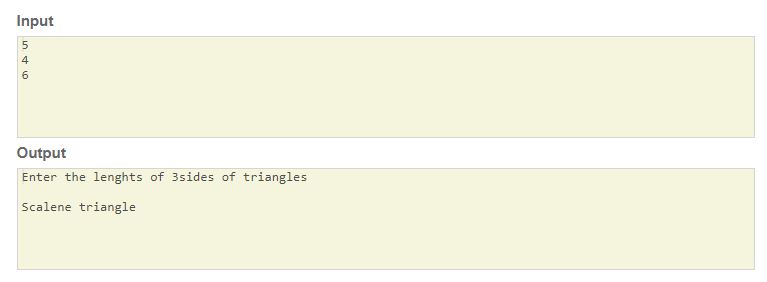