145. JAVA Program to print name which occurred more than once.
How does this program work?
- This program is used to print name which occured more than one time using java.
- String Palindrome means if it remains same even after reversing the each character.
- A string said to be a palindrome if original string is equal to the reverse of the given string otherwise it is not a palindrome.
Here is the code
//To print name which occurred more than one time
import java.util.Scanner;public class Name
{
public static void main(String[] args )
{
String string;
int count;
System.out.println("Enter a string:");
Scanner obj= new Scanner(System.in);
string=obj. nextLine();
//Converts the string into lowercase
string = string.toLowerCase();//Split the string into words using built-in function
String words[] = string.split(" ");System.out.println("Duplicate words in a given string : ");
for (int i = 0; i < words.length; i++)
{
count = 1;
for (int j = i+1; j < words.length; j++)
{
if (words[i].equals(words[j]))
{
count++;
//Set words[j] to 0 to avoid printing visited word
words[j] = "0"; }}
//Displays the duplicate word if count is greater than 1
if (count > 1 && words[i] != "0")System.out.println(words[i]);
}
}
}
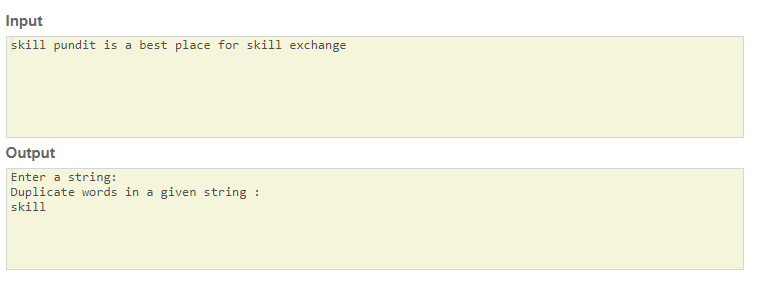