28. JAVA Program to find the roots of the given Quadratic Equation.
How does this program work?
- In this program you are going to learn about how to find roots of the given quadratic equation using java.
-
The term b2-4ac is known as the discriminant of a quadratic equation. The discriminant tells the nature of the roots.
- If discriminant is greater than 0, the roots are real and different.
- If discriminant is equal to 0, the roots are real and equal.
- If discriminant is less than 0, the roots are complex and different.
- In this program, library function sqrt() is used to find the square root of a number.
Here is the code
//To find the roots of the given Quadratic Equation
import java.util.Scanner;public class Root
{
public static void main(String[] args )
{
double a, b, c;
double root1, root2, imaginary, discriminant;
System.out.print("Enter the Values of a, b, c of Quadratic Equation : ");
Scanner sc=new Scanner(System.in);
a = sc.nextDouble();
b = sc.nextDouble();
c = sc.nextDouble();
discriminant = (b * b) - (4 * a *c);
if ( discriminant > 0 )
{
root1 = ( -b + Math.sqrt ( discriminant ) / ( 2 * a ));
root2 = ( -b - Math.sqrt ( discriminant ) / ( 2 * a ));
System.out.println("\n Two Distinct Real Roots Exists: \nroot1 = " + root1 + " and root2 = " + root2);
}
else
if ( discriminant == 0 )
{
root1 = root2 = -b / (2 * a);
System.out.println("\n Two Equal and Real Roots Exists:\n root1 = " + root1 + " and root2 = " + root2);
}
else
if ( discriminant < 0 )
{
root1 = root2 = -b / ( 2 * a );
imaginary =Math.sqrt ( -discriminant ) / ( 2 * a );
System.out.println("\n Two Distinct Complex Roots Exists:\n root1 = " + root1 + " + " + imaginary + " and root2 = " + root2 +" - " +imaginary);
}
}
}
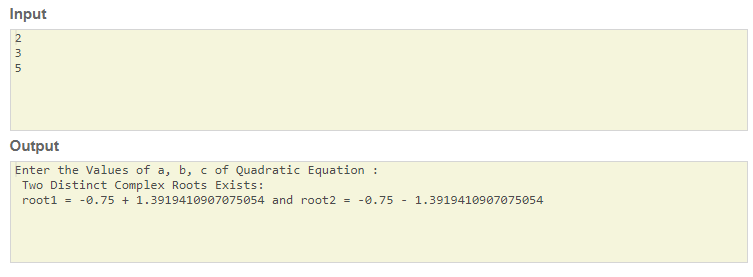