119. JAVA Program to find Subtraction of two matrices.
How does this program work?
- In this program we are going to learn about how to find Subtraction of two matrices using java.
- If rows and columns of given two matrices are same then only subtraction will be possible. Otherwise It is not possible to subtract a 2 × 3 matrix with a 3 × 2 matrix.
- Subtraction can be performed by corresponding their elements then result will be displayed on matrix format.
Here is the code
//Subtraction of two matrices
import java.util.Scanner;public class sub
{
public static void main(String args[] )
{
int m, n, i, j;
System.out.println("Enter the number of rows and columns of matrix:");
Scanner in = new Scanner(System.in );
m = in.nextInt();
n = in.nextInt();
int first[][] = new int[m][n];
int second[][] = new int[m][n];
int sum[][] = new int[m][n];
System.out.println("Enter the elements of first matrix:");
for (i = 0; i < m; i++)
for (j = 0; j < n; j++)
first[i] [j] = in.nextInt();
System.out.println("Enter the elements of second matrix:");
for (i = 0 ; i < m; i++)
for (j = 0 ; j < n; j++)
second[i] [j] = in.nextInt();
for (i = 0; i < m; i++)
for (j= 0; j< n; j++)
sum[i][j] = first[i][j] - second[i][j];
System.out.println("subtraction of two matrices:");
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
System.out.print(sum[i][j] + "\t" );
System.out.println();
}
}
}
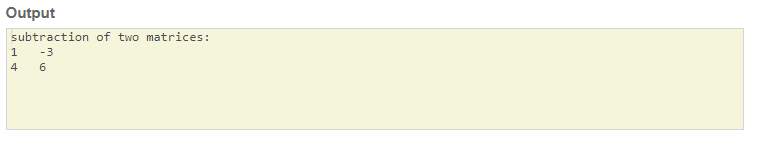