141. JAVA Program to Check given String is Palindrome or Not.
How does this program work?
- This program is used to check given string is palindrome or not using java.
- Palindrome String is a string which means if it remains same even after reversing the given string.
- A string said to be a palindrome if original string is equal to the reverse of the given string otherwise it is not a palindrome.
Here is the code
//To check given string is palindrome or not
import java.util.Scanner;public class palindrome
{
public static void main(String args[] )
{
String str, rev = "";
System.out.println("Enter a string:" );
Scanner sc= new Scanner(System.in );
str = sc.nextLine();
int length = str.length();
for ( int i = length - 1; i >= 0; i-- )
rev = rev + str.charAt(i);
if (str.equals(rev))
{
System.out.println(str+" is a palindrome");
}
else
{
System.out.println(str+" is not a palindrome");
}
}
}
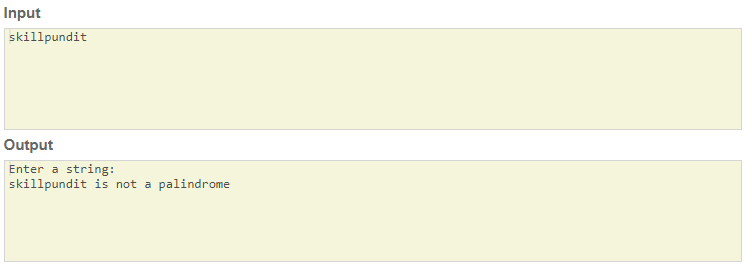