11. JavaScript Program to Calculate Monthly Income of a Person.
How does this program work?
- In this program you are going to learn about how to calculate Monthly Income of a Person following given Sales Commission Schedule using JavaScript.
- Here we are using if and nested if..else statements.
- After that By using the given Conditions Monthly income will be Calculated.
Here is the code
<html>
<head>
<title>JavaScript Program to calculate Monthly Income of a Person</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter income"/> </td>
</tr>
<tr>
<td> <button onclick="income ()">Submit</button> </td>
</tr>
</table>
<div id="num"></div>
</body>
<script type="text/javascript">
function income()
{
var income, com;
income = parseInt(document.getElementById ("first").value);
com = 0;
if(income >= 50000)
{
com = 375 + (income * 16)/100;
}
else
if (income <= 50000 && income >= 40000)
{
com = 370 + (income * 14)/100
}
else
if (income <= 40000 && income >= 30000)
{
com = 325 + (income * 12)/100
}
else
if (income <= 30000 && income >= 20000)
{
com = 300 + (income * 9)/100
}
else
if (income <= 20000 && income >= 10000)
{
com = 250 + (income * 5)/100
}
else
if (income <= 10000)
{
com = 200 + (income * 3)/100
}
document.getElementById ('num').innerHTML = "Commission : "+com;
}
</script>
</html>
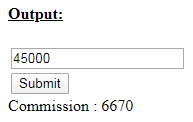
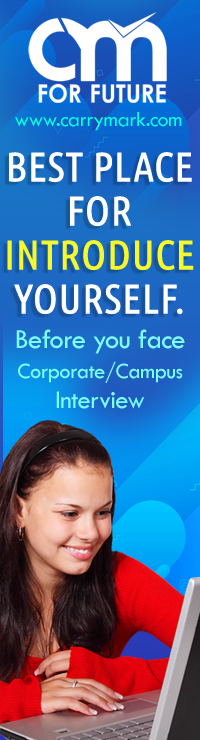