10. JavaScript Program to find the Biggest of given three numbers.
How does this program work?
- In this program you are going to learn about how to find out the largest number among given three numbers using javascript.
- This problem is solved by using nested if...else statement and &&(and) operator.
- After that by using given logic we get the Output.
Here is the code
<html>
<head>
<title>JavaScript program to find out the biggest of given three numbers</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter first number"/> </td>
</tr>
<tr>
<td> <input type="text" name="b" id="second" placeholder="Enter second number"/> </td>
</tr>
<tr>
<td> <input type="text" name="c" id="third" placeholder="Enter third number"/> </td>
</tr>
<tr>
<td> <button onclick = "biggest()">Submit</button> </td>
</tr>
</table>
<div id = "num"></div>
</body>
<script type="text/javascript">
function biggest()
{
var a,b,c;
a = parseInt(document.getElementById ("first").value);
b = parseInt(document.getElementById ("second").value);
c = parseInt(document.getElementById ("third").value);
if(a > b && a > c)
{
document.getElementById ("num").innerHTML = a+" is the biggest number";
}
else
if(b > c && b > a)
{
document.getElementById ("num").innerHTML = b+" is the biggest number";
}
else
{
document.getElementById ("num").innerHTML = c+"is the biggest number";
}
}
</script>
</html>
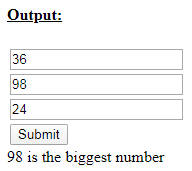
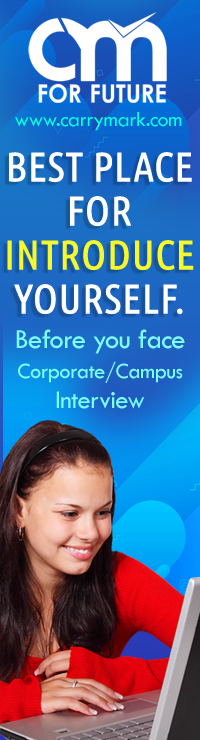