9. JavaScript Program to find Smallest of given two numbers.
How does this program work?
- In this program you are going to learn about how to find Smallest of given two numbers using JavaScript.
- In this program compare two numbers by using if statement, then we get the Smallest number among two numbers.
Here is the code
<html>
<head>
<title>JavaScript Program to find out the Smallest of given two numbers</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter first number"/> </td>
</tr>
<tr>
<td> <input type="text" name="b" id="second" placeholder="Enter second number"/> </td>
</tr>
<tr>
<td> <button onclick = "small()" >Submit</button> </td>
</tr>
</table>
<div id = "num"></div>
</body>
<script type="text/javascript">
function small()
{
var a,b;
a = parseInt(document.getElementById ("first").value);
b = parseInt(document.getElementById ("second").value);
if(a < b)
{
document.getElementById ("num").innerHTML = a+" is the Smallest number";
}
else
if(a > b)
{
document.getElementById ("num").innerHTML = b+" is the Smallest number";
}
else
{
document.getElementById ("num").innerHTML = "Both are equal";
}
}
</script>
</html>
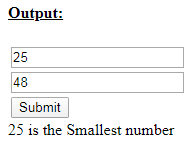
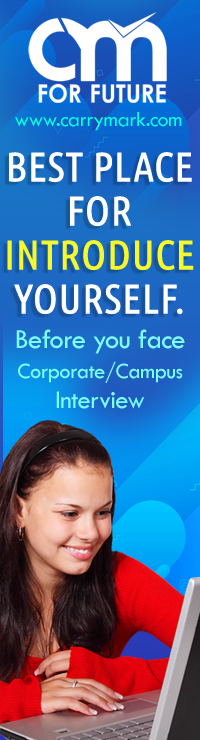