8. JavaScript Program to find the Distance between given two points.
How does this program work?
- In this program you will learn about how to find the distance between two points by using given X, Y co-odinates.
- First we need to store user entered values in x1, y1 and x2, y2.
- After that by using the given formula we can get the distance between two points by using JavaScript.
Here is the code
<html>
<head>
<title>JavaScript program to find the distance between two points</title>
</head>
<body>
<table>
<tr>
<td> <input type="text" name="a" id="first" placeholder="Enter x1 value"/> </td>
</tr>
<tr>
<td> <input type="text" name="b" id="second" placeholder="Enter y1 value"/> </td>
</tr>
<tr>
<td> <input type="text" name="c" id="third" placeholder="Enter x2 value"/> </td>
</tr>
<tr>
<td> <input type="text" name="d" id="fourth" placeholder="Enter y2 value"/> </td>
</tr>
<tr>
<td> <button onclick = "distance()">Submit</button> </td>
</tr>
</table>
<div id="num"></div>
</body>
<script type="text/javascript">
function distance()
{
var x, y, x1, x2, y1, y2, distance;
x1 = parseInt(document.getElementById("first").value);
y1 = parseInt(document.getElementById("second").value);
x2 = parseInt(document.getElementById("third").value);
y2 = parseInt(document.getElementById("fourth").value);
//Distance between two points (D = sqrt((x2-x1)^2 + (y2-y1)^2))
x = x2-x1;
y = y2-y1;
distance = Math.sqrt((x*x) + (y*y));
document.getElementById ('num').innerHTML ="Distance between two points is : "+distance;
}
</script>
</html>
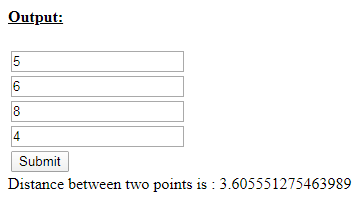
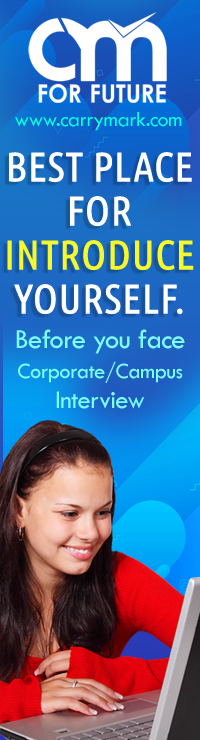