7. JavaScript Program to find Mechanical Energy of a particle is given by e=mgh+(1/2)mv^2.
How does this program work?
- In this programme you will learn about how to calculate Mechanical Energy using JavaScript.
- This programme requires the user inputs Mass ,displacement and velocity of the body.
- In this programme First we calculate Potential Energy and Kinetic Energy.
- And then perform mathematical calculation and display the output.
Here is the code
<html>
<head>
<title>JavaScript program to find Mechanical energy of a particle</title>
</head>
<body>
<table>
<tr>
<td>
<input type="text" name="a" id="first" placeholder="Enter Mass of an object(Kg)"/>
</td>
</tr>
<tr>
<td>
<input type="text" name="b" id="second" placeholder="Enter displacement of an object(m) "/>
</td>
</tr>
<tr>
<td>
<input type="text" name="c" id="third" placeholder="Enter velocity of an object(m/s) "/>
</td>
</tr>
<tr>
<td> <button onclick="mechanical_energy()" >Submit</button> </td>
</tr>
</table>
<div id = "num"></div>
<div id = "num1"></div>
<div id = "num2"></div>
</body>
<script type="text/javascript">
function mechanical_energy()
{
var n,potential_energy, kinetic_energy;
m = document.getElementById ("first").value;
h = document.getElementById ("second").value;
v = document.getElementById ("third").value;
var g = 9.8;
potential_energy = m*g*h; // Potential energy(P.E = mgh)
kinetic_energy = (0.5)*m*v*v; // Kinetic energy(K.E = 1/2mv^2)
//To calculate Mechanical energy(M.E = P.E+K.E)
mechanical_energy = potential_energy + kinetic_energy;
document.getElementById ('num').innerHTML = "Potential energy : "+potential_energy+"J";
document.getElementById ('num1').innerHTML = "Kinetic energy : "+kinetic_energy+"J";
document.getElementById ('num2').innerHTML = "Mechanical energy : "+mechanical_energy+"J";
}
</script>
</html>
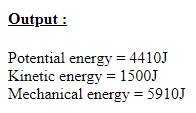
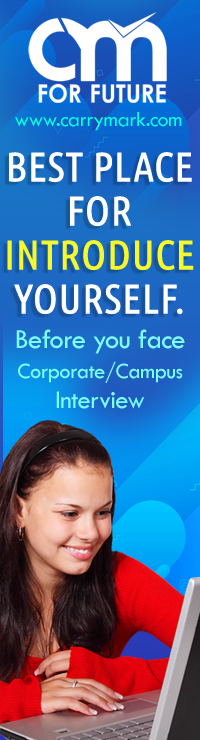