129. PHP Program to find Addition of two matrices.
How does this program work?
- In this program we are going to learn about how to find Addition of two matrices using PHP.
- If rows and columns of given two matrices are same then only addition will be possible. Otherwise It is not possible to add a 2 × 3 matrix with a 3 × 2 matrix.
- Addition can be performed by corresponding their elements then sum will be displayed on matrix format.
Here is the code
<html>
<head>
<title>PHP Program for Addition of two matrices</title>
</head>
<body>
<?php
// Elements of matrix a
$a = array
(
array(1, 0, 1),
array(4, 5, 6),
array(1, 2, 3)
);
//Elements of matrix b
$b = array
(
array(1, 1, 1),
array(2, 3, 1),
array(1, 5, 1)
);
// To determine the no.of rows and columns of given matrix
$rows = count($a);
$cols = count($a[0]);
//Performs addition of matrices a and b. Store the result in matrix sum
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++)
{
$sum[$i][$j] =0; //Initially sum to be declare with 0
$sum[$i][$j] = $a[$i][$j] + $b[$i][$j];
}
}
echo ("Addition of two matrices: <br>");
// To print result in matrix form
for($i = 0; $i < $rows; $i++)
{
for($j = 0; $j < $cols; $j++)
{
echo($sum[$i][$j] . " ");
}
echo("<br>" );
}
?>
</body>
</html>
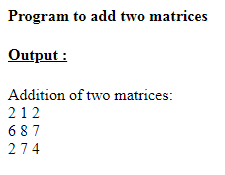
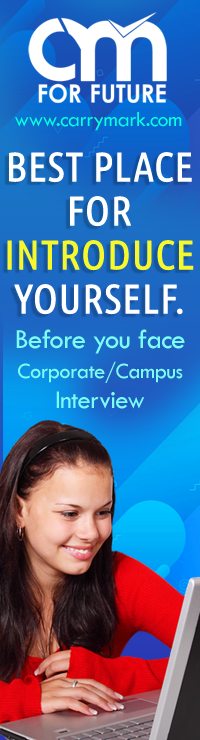