PHP Theory
PHP Variables
Variables are name of the memory location. It is used to store data.
Rules for defining variable
- A variable name must start with $ sign.
- A variable name can consist of alphabets, digits and the underscore character.
- Variable name can start with alphabet, and underscore. It can not start with a digit.
- Blank spaces cannot be used in variable names.
Creating a variable
The variable name start with dollar sing '$'.
Syntax |
---|
$variable_name = value; $txt = "Skill Pundit"; $txt = 10; $y = 10.5; |
PHP Variable Scope:
PHP has three types of variable scopes:
Local variable
The variable are declared inside a function is called local variable. That means it cannot access the outside that function.
Example |
---|
<?php function skill () { $x = 10; // local variable echo "The value of x inside function is : $x"; } skill(); echo "The value of x outside function is : $x"; ?> Output: The value of x inside function is : 10 The value of x outside function is : 10 |
Global variable
The variables are declared outside the function is called global variable. We use the 'global' keyword before a variable to access the global variable within a function.
Example |
---|
<?php $x = 10; // Global variable function skill () { echo "The value of x inside function is : $x"; } skill(); echo "The value of x outside function is : $x"; ?> Output: The value of x inside function is : 10 The value of x outside function is : 10 |
Using 'global' keyword
Example |
---|
<?php $x = 10; // Global variable function skill () { global $x; } skill(); echo "The value of x is : $x"; // Output: 10 ?> |
Static variable
Regularly when a function ends, the entirety of its variables free its values. At times we need to hold these values for the further activity. To do this 'static' keyword is used before the variables.
Example |
---|
<?php function skill () { $x = 1; echo $x; $x++; } skill(); echo " "; skill(); echo " "; skill(); ?> Output: 1 1 1 |
Using static keyword
Example |
---|
<?php function skill () { static $x = 1; echo $x; $x = 1; } skill(); echo " "; skill(); echo " "; skill(); ?> Output: 1 2 3 |
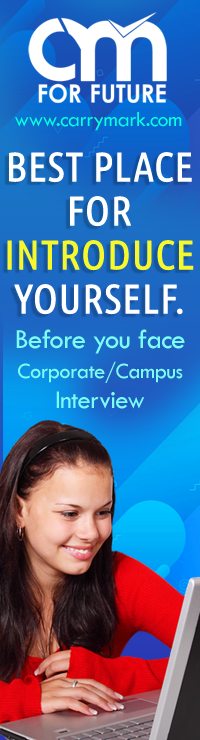