PHP Theory
Arrays in PHP:
An array is a collection(or blend) of elements. And it is used to store the multiple values in one variable. Each value stored in an array is called an element of the array.
Types of Arrays in PHP
- Indexed arrays - An array with numeric index
- Associative arrays - An array with named keys
- Multidimensional arrays - An array containing one or more arrays
How to create an array in PHP
Syntax |
---|
$skill = array( values ); |
Indexed arrays
An indexed arrays or Numeric arrays are used to store any type of elements with numberic index. The numeric index is starts from zero. There are two methods to create indexed arrays
Example |
---|
First method: $skillpundit = array("C", "Java", "PHP","Python"); |
Example |
---|
Second method:
$skillpundit[0] = "C"; $skillpundit[1] = "Java"; $skillpundit[2] = "PHP"; $skillpundit[3] = "Python"; |
Associative arrays
In associative array, the keys assigned to values.
Example | |
---|---|
$salary= array("Gowtham" =>15000, "Arjun" =>35000, "John" =>50000); $salary["Gowtham"] = "15000"; $salary["Arjun"] = "35000"; $salary["John"] = "50000"; |
Multidimensional arrays
A multi-dimensional array each element within the primary array can also be an array.
Example |
---|
$favorites = array( array( "name" => "John", "course" => "Python" , ), array( "name" => "Ram", "course" => "Java", ), array( "name" => "Peter", "course" => "Android", ) ); |
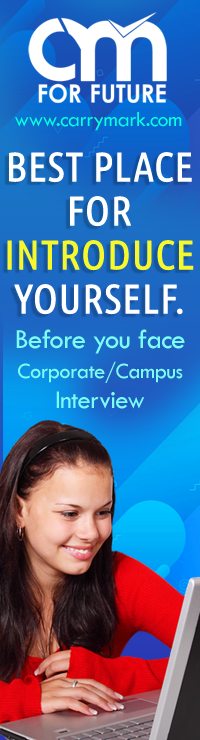