5. PHP Program to find Area and Perimeter of a Square.
How does this program work?
- In this program you will learn about how to calculate Area and Perimeter of a Square using PHP.
- To calculate the perimeter and area of a Square side of the square is required.
- This program performs mathematical calculations and displays output.
Here is the code
<html>
<head>
<title>PHP Program To find Area and Perimeter of a Square</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter length of square side"/>
</td> </tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/></td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$a = $_POST['num1'];
$area = $a * $a; //Equation for Area of a square
$perimeter = 4 * $a; //Equation for Perimeter of a square
echo "Area of square " .$a." is "." = ".$area ;
echo "Perimeter of square " .$a." is "." = ".$perimeter;
return 0;
}
?>
</body>
</html>
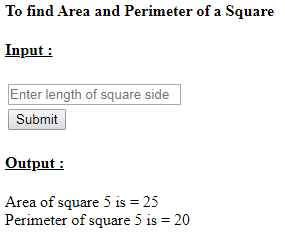
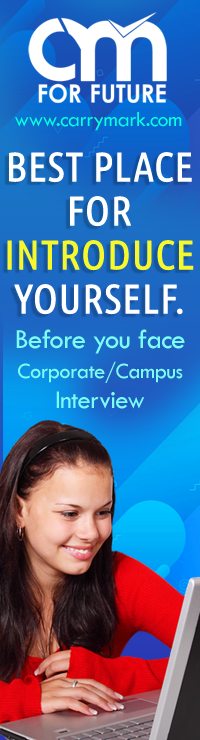