143. PHP Program to check given matrix is Symmetric or not.
How does this program work?
- In this program we are going to learn about how to check given matrix is Symmetric or not using PHP.
- Symmetric matrix means Original matrix and Transpose of the given matrix should be in equal.
- Find the Transpose of the given matrix.
- After that to check these two matrices are equal or not, If these are equal it is Symmetric else it is not Symmetric matrix.
Here is the code
<html>
<head>
<title>PHP Program To check given matrix is Symmetric or not </title>
</head>
<body>
<?php
// Elements of matrix a
$a = array
(
array(1, 3, 5),
array(3, 2, 4),
array(5, 4, 1)
);
$rows = count($a);
$cols = count($a[0]);
// To find the transpose of the given matrix
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++) {
$t[$i][$j] = $a[$j][$i];
}
}
// To print original matrix
echo("Original matrix is: <br>");
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++) {
echo ($a[$i][$j] . " ");
}
echo("<br>");
}
//To print transpose matrix
echo("Transpose of given matrix is:<br>");
for($i = 0; $i < $cols; $i++) {
for($j = 0; $j < $rows; $j++) {
echo($t[$i][$j] . " ");
}
echo("<br>");
}
//To check Original matrix and Transpose matrix are equal or not
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++) {
if($a[$i][$j] != $t[$i][$j])
{
echo "Given matrix is not a Symmetric";
exit(0);
}
}
}
echo "Given matrix is a Symmetric";
?>
</body>
</html>
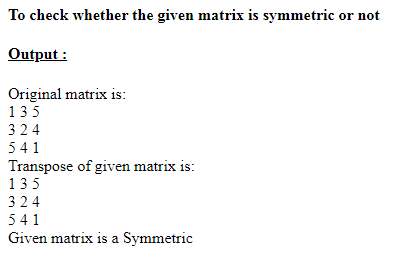
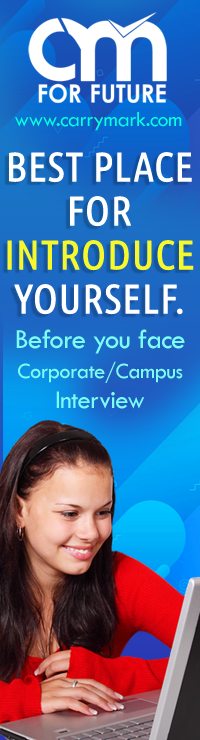