60. PHP Program to find the given number is Armstrong or Not.
How does this program work?
- In this program you are going to learn about to check if a number is Armstrong number or not using PHP.
- An Armstrong number is a number whose value is equal to the sum of the cubes of its digits.
- If sum is equal to the original number, then that number is armstrong number else the number is not a armstrong number.
Here is the code
<html>
<head>
<title>PHP Program To Check a given number is Armstrong number or Not</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter a number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$n = $_POST['num'];
$x = $n;
$r = 0;
$sum = 0;
while($n>1)
{
$r = $n%10;
$sum = $sum+($r*$r*$r);
$n =$n/10;
}
if($x==$sum)
{
echo "$x is Amstrong number";
}
else
{
echo "$x is not a Amstrong number";
}
}
return 0;
?>
</body>
</html>
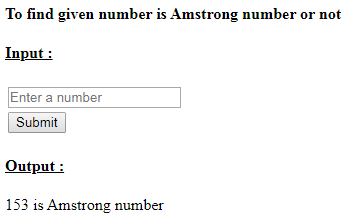
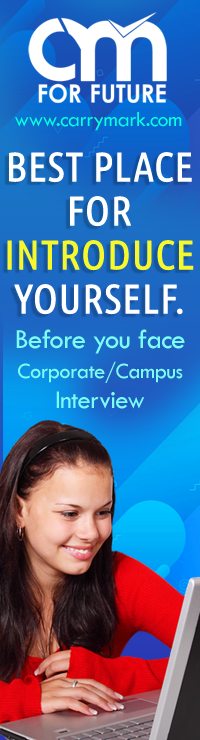