122. PHP Program to Compute the Cosine Series 1 - x^2/2! + x^4/4! – x^6/6! + …… x^n/n!
How does this program work?
- This program is used to find the Sum of the given Cosine series 1 - x^2/2! + x^4/4! – x^6/6! + …… x^n/n! using PHP.
- The integer entered by the user is stored in variable x and n but x value in degrees.
- By using the for loop condition we can easily calculate the sum of cosine series.
Here is the code
<html>
<head>
<title>Find the Sum of Cosine Series</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter x value in degrees"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter n value"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$x = $_POST['num1'];
$n = $_POST['num2'];
$x1 = $x;
$res= 1;
$fact = 1;
$pow = 1;
$sign = 1;
$pi = 3.142; //pi= 22/7
$precision = 3;
$x = $x * $pi/(180.0);
// Loop to calculate the value of Cosine
for ( $i = 1; $i < $n; $i++)
{
$sign = $sign *-1;
$fact = $fact * (2 * $i - 1) * (2 * $i);
$pow = $pow * $x * $x;
$res = $res + $sign * $pow / $fact;
}
echo "X value : ".$x1."</br>";
echo "N value : ".$n."</br>";
echo " The value of Cos($x) is : " .number_format($res, $precision);
return 0;
}
?>
</body>
</html>
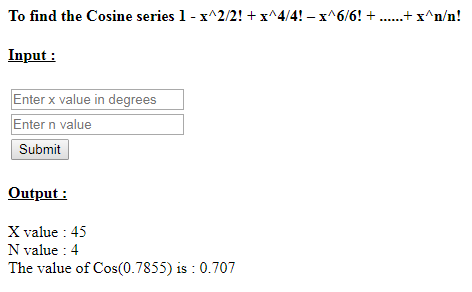
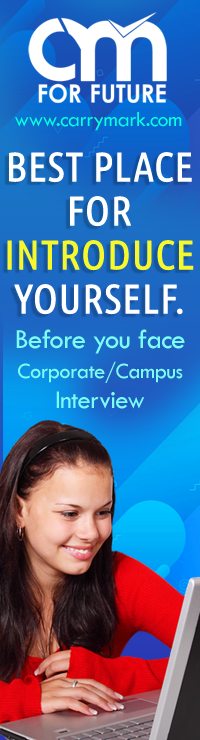