79. PHP Program to Convert Decimal number into Binary number.
How does this program work?
- This program is used to convert given Decimal number into equivalent of Binary number using PHP.
- That means convert number with base value 10 to base value 2.
- The integer entered by user will store in one variable. Divide that number by 2.
- Store the remainder when the number is divided by 2 in an array, Repeat the above step until the number is greater than zero.
Here is the code
<html>
<head>
<title>PHP Program To convert Decimal number into Binary number</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter decimal number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$n = $_POST['num'];
$num = $n;
$n1 = $n;
$binary;
$i = 0; //Count for binary array
while ($n > 0)
{
$binary[$i] = $n % 2; //Remainder will stored in binary array
$n = (int)($n / 2);
$i++; //Count increment
}
echo "The Decimal number is : ".$num ;
echo "The Equivalent Binary number is : ";
for ($j = $i - 1; $j >= 0; $j--)
echo $binary[$j];
return 0;
}
?>
</body>
</html>
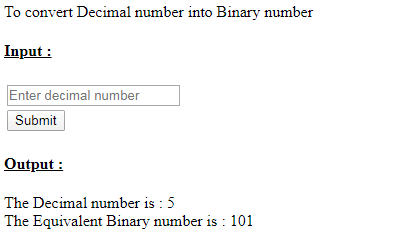
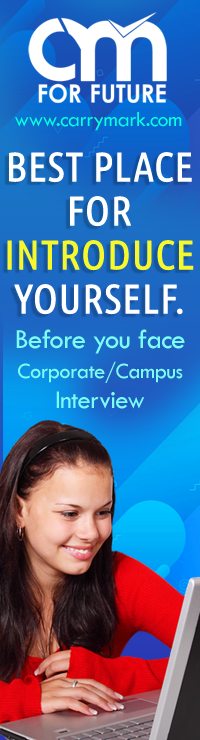