166. PHP Program to Convert Lowercase text into Uppercase text.
How does this program work?
- This program is used to convert lowercase text into uppercase text using PHP.
Here is the code
<html>
<head>
<title>PHP Program To convert all lowercase into uppercase</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="string" value="" placeholder="Enter a string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
function Upper ($str) {
$var = str_split($str); // conversion of string into array
$arrlength = count ($var); //counting lengh of array
$arr = array();
for($i = 0; $i < $arrlength; $i++)
{
$arr[$i] = ord($var[$i]);
}
for($x = 0; $x < $arrlength; $x++)
{
//To Convert lowercase into uppercase
if($arr[$x]>=97 && $arr[$x]<=122) {
$arr[$x] = $arr[$x]-32;
}
}
for($j = 0; $j < $arrlength; $j++)
{
$var[$j] = chr($arr[$j]);
}
$name = implode ("",$var);
// conversion of array into string
echo $name; // printing string
}
if(isset($_POST['submit'])) {
$str = $_POST['string'];
echo "Input string is : " . $str."</br>";
echo "String in Upper case : ";
Upper( $str);
return 0;
}
?>
</body>
</html>
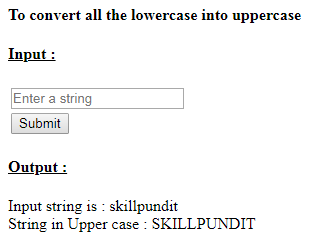
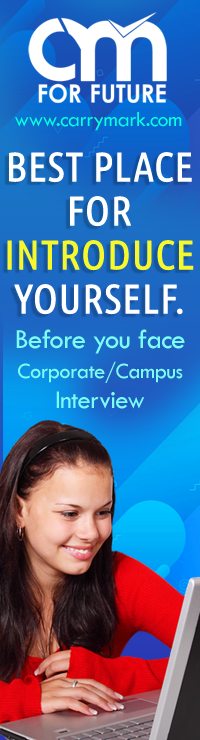