149. PHP Program to find the minimum and maximum numbers of given numbers using recursion.
How does this program work?
- In this program you will learn about how to find the minimum and maximum numbers of given numbers recursively in PHP.
Here is the code
<html>
<head>
<title>PHP Program To find the minimum and maximum numbers of given numbers recursively</title>
</head>
<body>
<?php
// Returns maximum in array
function getMax($array)
{
$n = count($array);
$max = $array[0];
for ($i = 1; $i < $n; $i++)
if ($max < $array[$i])
$max = $array[$i];
return $max;
}
// Returns maximum in array
function getMin($array)
{
$n = count($array);
$min = $array[0];
for ( $i = 1; $i < $n; $i++)
if ($min > $array[$i])
$min =$array[$i];
return $min;
}
$array = array(1, 2, 3, 4, 5);
echo "Maximum number is: ".(getMax($array))."<br>";
echo("\n");
echo"Minimum number is: ".(getMin($array));
?>
</body>
</html>
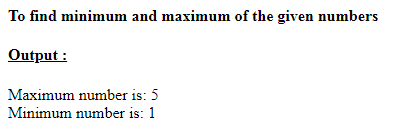
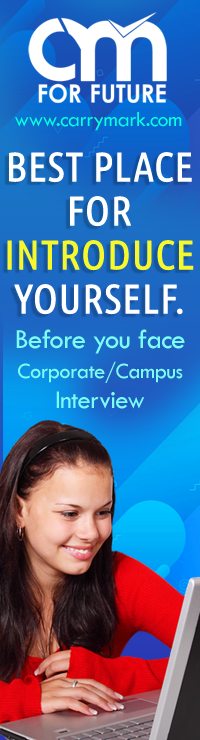