169. PHP Program to Insert a word at exact position in given text.
How does this program work?
- This program is used to insert a word in given text at exact positon using PHP.
- By using if condition we can find the number of vowels, consonants and whitespaces from given text.
Here is the code
<html>
<head>
<title>PHP Program To Insert a word in main string at exact position</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter string"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter insert string to add"/> </td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter the position where Word has to be inserted"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit'])) {
$str1 = $_POST[ 'num1'];
$str2 = $_POST[ 'num2'];
$pos = $_POST[ 'num3'];
echo "Original String: ".$str1."</br>";
echo "Insert String: ".$str2."</br>";
echo "Position of insert: ".$pos."</br>";
echo substr_replace( $str1, $str2, $pos, 0 );
return 0;
}
?>
</body>
</html>
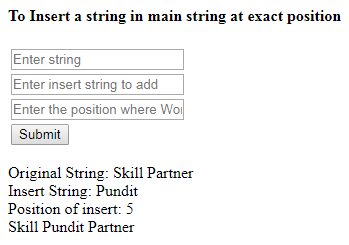
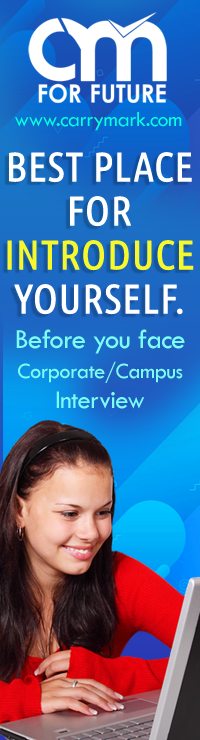