56. PHP Program to find Numbers Divisible by both 3 and 7 from 1 to 100 numbers.
How does this program work?
- In this program we are going to learn about how to find the numbers which are divisible by both 3 and 7 from 1 to 100 numbers using PHP.
- When the number is divided by both 3 and 7, we use the remainder operator % to compute the remainder.
- If the remainder is zero, then that number is divisible of both 3 and 7.
- After doing this for each value, then we get the numbers from 1 to 100.
Here is the code
<html>
<head>
<title>PHP Program To print the numbers which are divisible by both 3 and 7 from 1 to 100</title>
</head>
<body>
<?php
echo (" The numbers which are divisible by both 3 and 7 from 1 to 100 : \n")." ";
for($i = 1; $i <= 100; $i++)
{
// Condition to check divison of 3 and 7
if( ($i%3) == 0 && ($i%7) == 0)
{
echo $i;
}
}
return 0;
?>
</body>
</html>
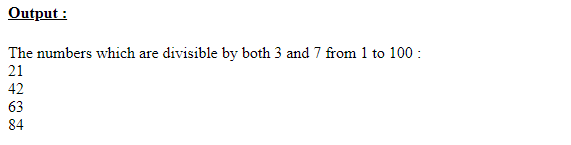
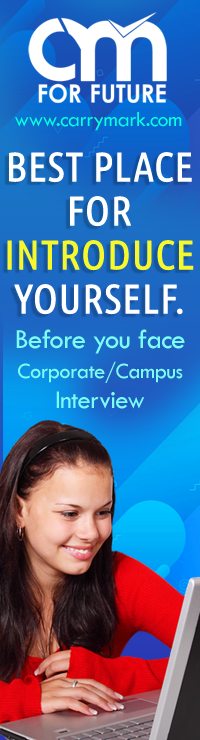