137. PHP Program to print Inner Square elements of a given matrix.
How does this program work?
- In this program we are going to learn about how to print Inner Square elements of a given matrix using PHP.
- Elements are stored in array variable. Here we used 4*4 matrix to display inner square elements of given matrix.
Here is the code
<html>
<head>
<title>PHP Program To print Inner Square of a given matrix</title>
</head>
<body>
<?php
//Initialize A matrix
$a =array
(
array( 1, 2, 3, 4 ),
array( 2, 3, 4, 1 ),
array( 3, 4, 2, 1 ),
array( 4, 3, 2, 1 )
);
$n = count ($a);
echo("Original matrix is: <br>"); // to print Original matrix
for($i = 0; $i < $n; $i++) {
for($j = 0; $j < $n; $j++) {
echo ($a[$i][$j] . " ");
}
echo("<br>");
}
echo "Inner square of the given matrix is :"."<br>";
function inner($a, $m, $n)
{
for ($i = 1; $i < $m; $i++)
{
for ($j = 1; $j < $n-1; $j++)
{
if ( $i == 1)
echo $a[$i][$j], " ";
else if ($i == $m /2)
echo $a[$i][$j], " ";
else
echo " ". " ";
}
echo "<br>";
}
}
inner($a, 4, 4);
?>
</body>
</html>
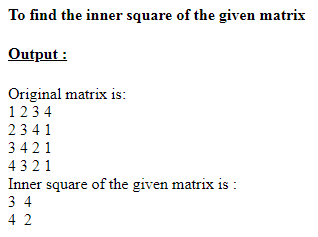
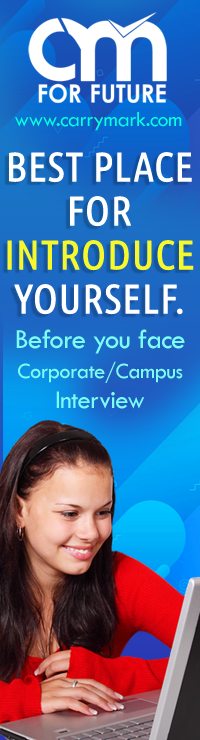