188. PHP Program to find the Reverse of a given string.
How does this program work?
- This program is used to find the Reverse of a given string using PHP.
- The string entered by the user is stored in variable str.
- By using if condition we can find the reverse of a given string.
Here is the code
<html>
<head>
<title>PHP Program To find the Reverse of a given string</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="name1" value="" placeholder="Enter string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
function reverse($str)
{
if (($str == null) || (strlen($str) <= 1))
echo ($str);
else
{
echo ( $str[strlen($str) - 1] );
reverse(substr($str, 0, (strlen($str) - 1)));
}
}
if(isset($_POST['submit'])) {
$str = $_POST['name1'];
echo "Original string: " . $str."</br>";
//Displays the reverse of given string
echo"Reverse of given string: " ;
reverse($str);
}
?>
</body>
</html>
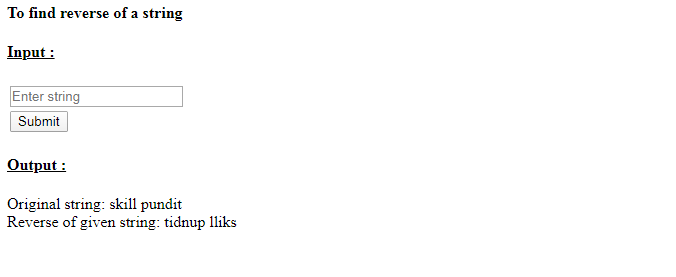
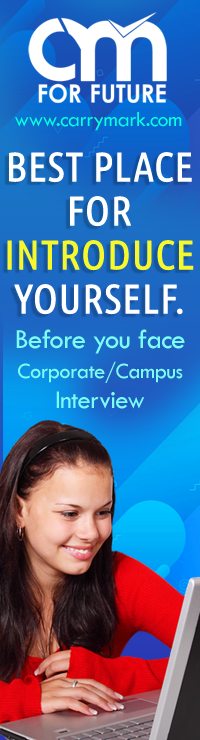