84. PHP Program to Sort the elements in Descending Order.
How does this program work?
- This program is used to Sort the elements in Descending Order using PHP.
- In this program, an array of elements can be sorted without using any sorting function in PHP.
- Each element is compared with the next element, If the element is less than the other in comparison then the elements are swapped.
- After doing this for each element of the given array, then we get the elements in descending order.
Here is the code
<html>
<head>
<title>PHP Program To arrange the elements in Descending Order</title>
</head>
<body>
<?php
$array =array(9, 2, 18, 34, 3, 10, 15);
// Get the size of array
$count = count($array);
echo "Before Sorting of Array elements: ";
// Print array elements before sorting
foreach ($array as $value) {
echo $value. " ";
}
for ($i = 0; $i < $count; $i++) {
for ($j = $i + 1; $j < $count; $j++) {
if ($array[$i] < $array[$j]) {
$temp = $array[$i];
$array[$i] = $array[$j];
$array[$j] = $temp;
}
}
}
echo "After sorting of Array elements:" ";
// Print array elements after sorting
for($i=0; $i<$count;$i++)
{
echo $array[$i]." ";
}
?>
</body>
</html>
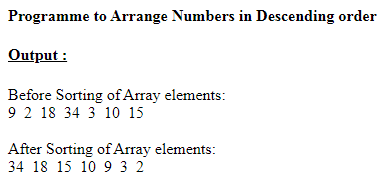
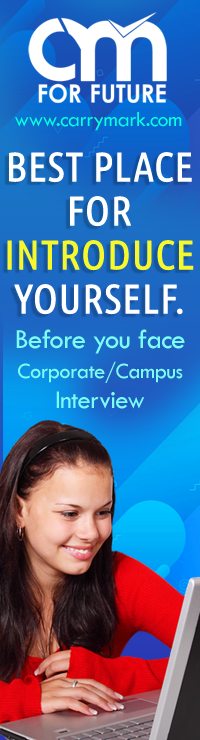