181. PHP Program to store student details in records and print it.
How does this program work?
- In this program you are going to learn about how to store student details in records and print it using PHP.
- By using functions we can read the student details and print it.
Here is the code
<?php
if(isset($_POST['submit'])) {
$size = array();
$size = $_POST['Fname'];
$size1 = $_POST['Froll'];
$size2 = $_POST['Fdob'];
echo "<table>\n";
echo "<tr><th> Name </th><th> Rollno </th><th> DateOfBirth </th></tr>\n";
foreach($size as $key=>$value)
{
echo "<tr>";
echo "<td>" . $value . "</td>\n";
echo "<td>" . $_POST['Froll'][$key] . "</td>\n";
echo "<td>" . $_POST['Fdob'][$key] . "</td>\n";
echo "</tr>";
}
echo "</table>";
return 0;
}
?>
<html>
<head>
<title>PHP Program To store student details in records and print it</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" id="num1" name="num1" value="" placeholder="Enter array size"/> </td>
</tr>
<div id="screens">
</div>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script>
function create(param)
{
'use strict';
var i;
$("#screens").empty();
for (i = 0; i < param; i += 1)
{
$('#screens').append('<input type="text" name="Fname[]" placeholder="Enter name">');
$('#screens').append('<input type="text" name="Froll[]" placeholder="Enter Rollno">');
$('#screens').append('<input type="text" name="Fdob[]" placeholder="Enter dateofbirth">');
}
}
$('#num1').change(function ()
{
create($(this).val());
});
</script>
</html>
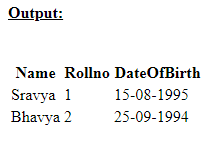
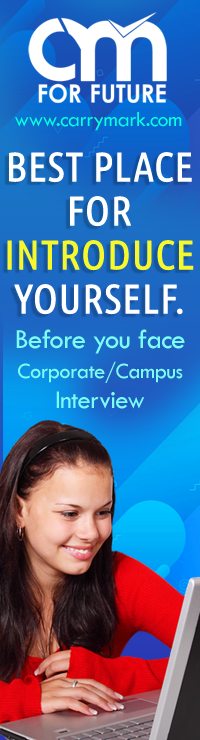