152. PHP Program to find Sum of given number upto single digit using recursion.
How does this program work?
- This program is used to find Sum of given number upto single digit using recursion in PHP.
Here is the code
<html>
<head>
<title>PHP Program To find the Sum of given number upto single digit recursively</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter any number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit'])) {
$num = $_POST['num1'];
$x = $num;
$sum = 0;
while($num > 0 || $sum > 9)
{
if($num == 0)
{
$num = $sum;
$sum = 0;
}
$sum += $num % 10;
$num = (int)$num / 10;
}
echo "Sum of digits of a number $x upto single digit answer is : " . $sum;
return 0;
}
?>
</body>
</html>
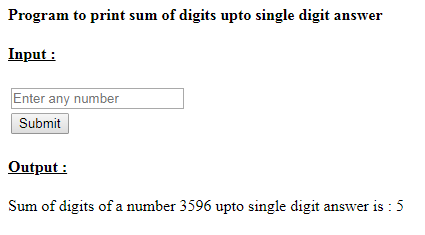
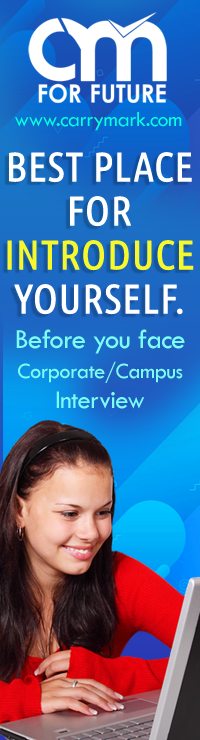