46. PHP Program to find the Sum of N Distinct numbers.
How does this program work?
- In this program we are going to learn about how to find the Sum of N even numbers using PHP.
- The integer entered by the user is stored in variable n.
- Declare variable sum to store the sum of distinct numbers and initialize it with 0.
- By using for loop we can add the sum of n distinct numbers.
Here is the code
<html>
<head>
<title>PHP Program To find the Sum of N Distinct numbers</title>
</head>
<body>
<?php
function sumOfDistinct($a, $n)
{
$sum = 0;
echo "The array elements are : ";
for ($i = 0; $i < $n; $i++)
{
// If element appears first time
if ( $a[abs($a[$i]) - 1] >= 0)
{
$sum += abs($a[$i]);
$a[abs($a[$i]) - 1] *=-1;
// To print the numbers after removing the duplicate numbers
echo abs($a[$i]);
}
}
return $sum;
}
$a = array(5, 1, 2, 4, 6, 7, 3, 6, 7);
$n = sizeof($a);
echo "Sum of n distinct numbers are: ".sumOfDistinct($a, $n) ;
return 0;
?>
</body>
</html>
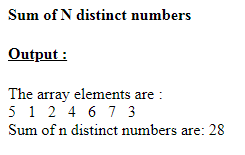
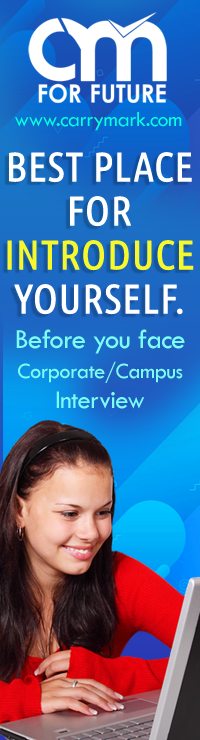