28. PHP Program to Check whether the given year is leap year or not.
How does this program work?
- In this program you are going to learn about to check if a year is leap year or not using PHP.
- In this program A year is leap if it is perfectly divisible by 4 and 400.
- When the year is divided by 4 and 400, we use the remainder operator % to compute the remainder.
- If the remainder is zero, the year is leap year else the year is not a leap year.
Here is the code
<html>
<head>
<title>PHP Program To Check a given year is leap year or not</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter a year"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$year = $_POST['num'];
if($year%400 == 0) // If Exactly divisible by 400 e.g. 1600, 2000
{
echo " $year is a leap year " ;
}
else if ($year%100 == 0) //If Exactly divisible by 100 and not by 400 e.g. 1900, 2100
{
echo " $year is not a leap year " ;
}
else if ($year%4 == 0) //If Exactly divisible by 4 and neither by 100 nor 400 e.g. 2020
{
echo " $year is a leap year " ;
}
else // Not divisible by 4 or 100 or 400 e.g. 2017, 2018, 2019
{
echo " $year is not a leap year " ;
}
return 0;
}
?>
</body>
</html>
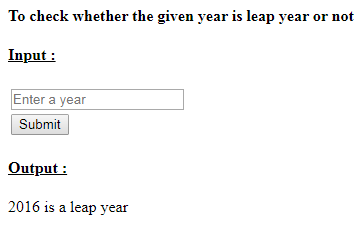
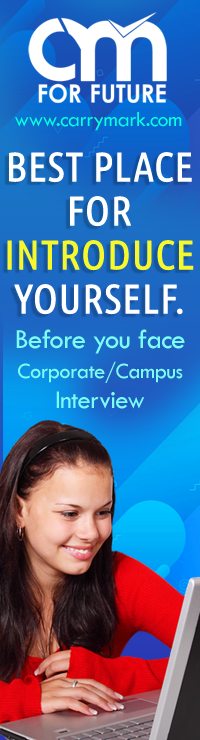