156. PHP Program to generate Fibonacci number using static.
How does this program work?
- This program is used to generate Fibonacci number using static in PHP.
- Fibonacci series means the previous two elements are added to get the next element starting with 0 and 1.
- First we initializing first and second number as 0 and 1, and print them.
- The third number will be the sum of the first two numbers by using loop.
Here is the code
<html>
<head>
<title>PHP Program To generate Fibonacci number using static</title>
</head>
<body>
<?php
//Intialise Variables
$n = 5;
$num1 = 0;
$num2 = 1;
echo "Fibonacci Series of $n is : <br>";
echo $num1.' '.$num2;
//loop to print Fibonacci series
for($i=2;$i<$n;$i++)
{
$num3 = $num1+$num2 ;
echo ' '.$num3 ;
$num1 =$num2 ;
$num2 = $num3;
}
return 0;
?>
</body>
</html>
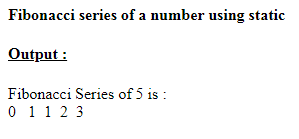
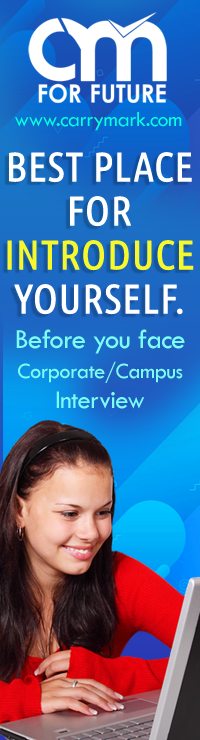