77. JAVA Program to Convert Decimal number into Binary number.
How does this program work?
- This program is used to convert given Decimal number into equivalent of Binary number using java.
- That means convert number with base value 10 to base value 2.
- The integer entered by user will store in one variable. Divide that number by 2.
- Store the remainder when the number is divided by 2 in an array, Repeat the above step until the number is greater than zero.
Here is the code
//Java Program - Decimal to Binary Conversion.//
import java.util.Scanner;public class Number
{
public static void main(String arg[])
{
int n;
System.out.println("Enter a decimal number");
Scanner skill=new Scanner(System.in );
n=skill.nextInt();
int bin[]=new int[100];
int i = 0;
while(n > 0)
{
bin[i++] = n%2;
n = n/2;
}
System.out.print("Binary number is: " );
for(int j = i-1;j >= 0;j--)
{
System.out.print(bin[j]);
}
}
}
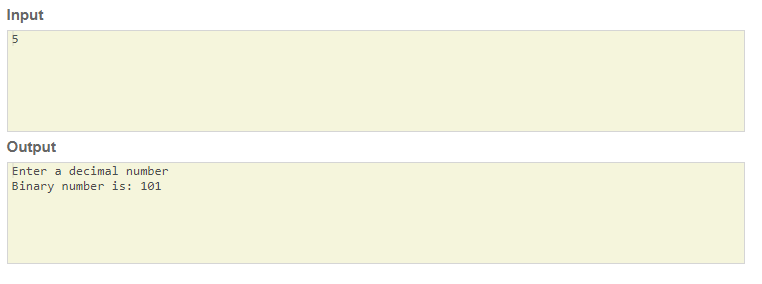