76. JAVA Program to Separate Zeros from the given Array elements.
How does this program work?
- This program is used to find the zeros from the given array elements using java.
Here is the code
//To separate zeros from the given array elements
import java.util.Arrays;import java.util.Scanner;
public class Array
{
public static void main(String args[] )
{
int size;
System.out.println("Enter the size of the array : ");
Scanner sc= new Scanner(System.in);
size = sc.nextInt();
int[] myArray = new int[size];
System.out.println("Enter the elements of the array: ");
for(int i=0; i< size; i++)
{
myArray[i] = sc.nextInt();
}
System.out.println("The Entered array is: "+Arrays.toString(myArray));
// System.out.println("Resultant array: ");
int pos = 0;for(int i=0; i< myArray.length; i++)
{
if(myArray[i]!=0)
{
myArray[pos]=myArray[i];
pos++;
}
}
while(pos< myArray.length)
{
myArray[pos] = 0;
pos++;
}
System.out.println("The array is: "+Arrays.toString(myArray));
}
}
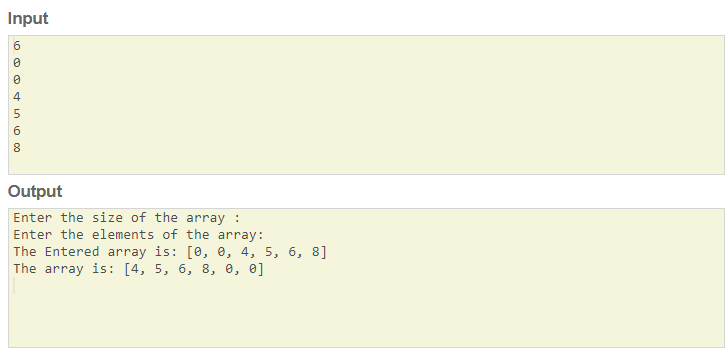