131. JAVA Program to find the Sum of n natural numbers using recursion.
How does this program work?
- In this program we are going to learn about how to find the Sum of n natural numbers using java.
- The integer entered by the user is stored in variable n.
- Declare variable sum to store the sum of numbers and initialize it with 0.
- By using Recursion method we can find the sum of n natural numbers.
Here is the code
//To find the Sum of N natural numbers using recursion
import java.util.Scanner;public class Recursion
{
public static void main(String args[] )
{
//variable declaration
int sum,num;//create a scanner object for input
System.out.print("Enter number: \n" );Scanner obj=new Scanner(System.in );
num=obj.nextInt();
sum=addnumbers(num );
System.out.print("Sum of natural numbers are:"+sum);
}
static int addnumbers(int num)
{
if (num!= 0)
return num+addnumbers(num-1);
else
return num;
}}
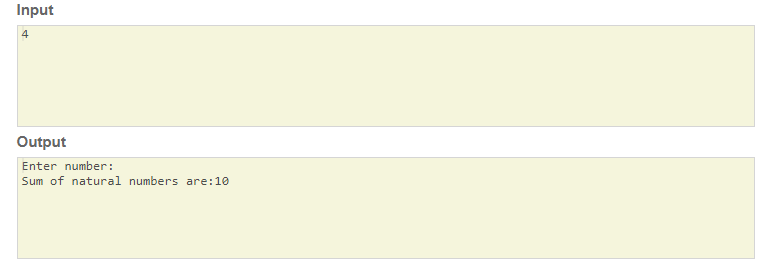